In the ever-evolving landscape of technology, data structures serve as the backbone of efficient programming and algorithm design. They are essential for organizing, managing, and storing data in a way that enables quick access and modification. Whether you’re a seasoned developer or a budding programmer, a solid understanding of data structures is crucial for tackling complex problems and optimizing performance.
As technical interviews become increasingly competitive, mastering data structures is not just an advantage—it’s a necessity. Employers often prioritize candidates who can demonstrate a deep understanding of these concepts, as they are fundamental to writing clean, efficient code. From arrays and linked lists to trees and graphs, the ability to choose the right data structure for a given problem can set you apart in the hiring process.
This comprehensive guide is designed to equip you with a robust collection of data structure interview questions that will challenge your knowledge and sharpen your problem-solving skills. You can expect to explore a variety of questions, ranging from basic concepts to more advanced scenarios, along with insights into best practices and common pitfalls. Whether you’re preparing for an upcoming interview or simply looking to enhance your understanding, this ultimate list will serve as a valuable resource on your journey to mastering data structures.
Basic Data Structure Questions
What is a Data Structure?
A data structure is a specialized format for organizing, processing, and storing data. It provides a way to manage large amounts of data efficiently, allowing for easy access and modification. Data structures are essential in computer science and programming as they enable developers to handle data in a way that optimizes performance and resource utilization.
At its core, a data structure defines the relationship between the data and the operations that can be performed on that data. For instance, a simple array allows for indexed access to its elements, while a linked list provides a way to traverse elements sequentially. The choice of data structure can significantly impact the efficiency of algorithms and the overall performance of applications.
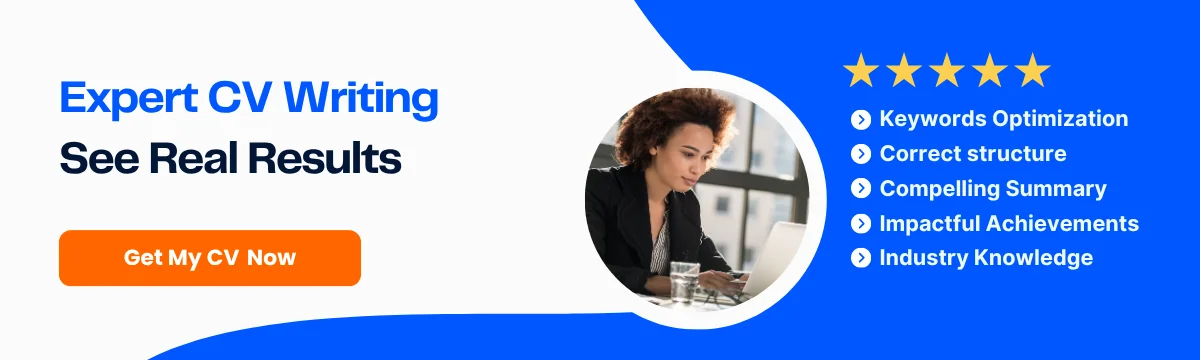
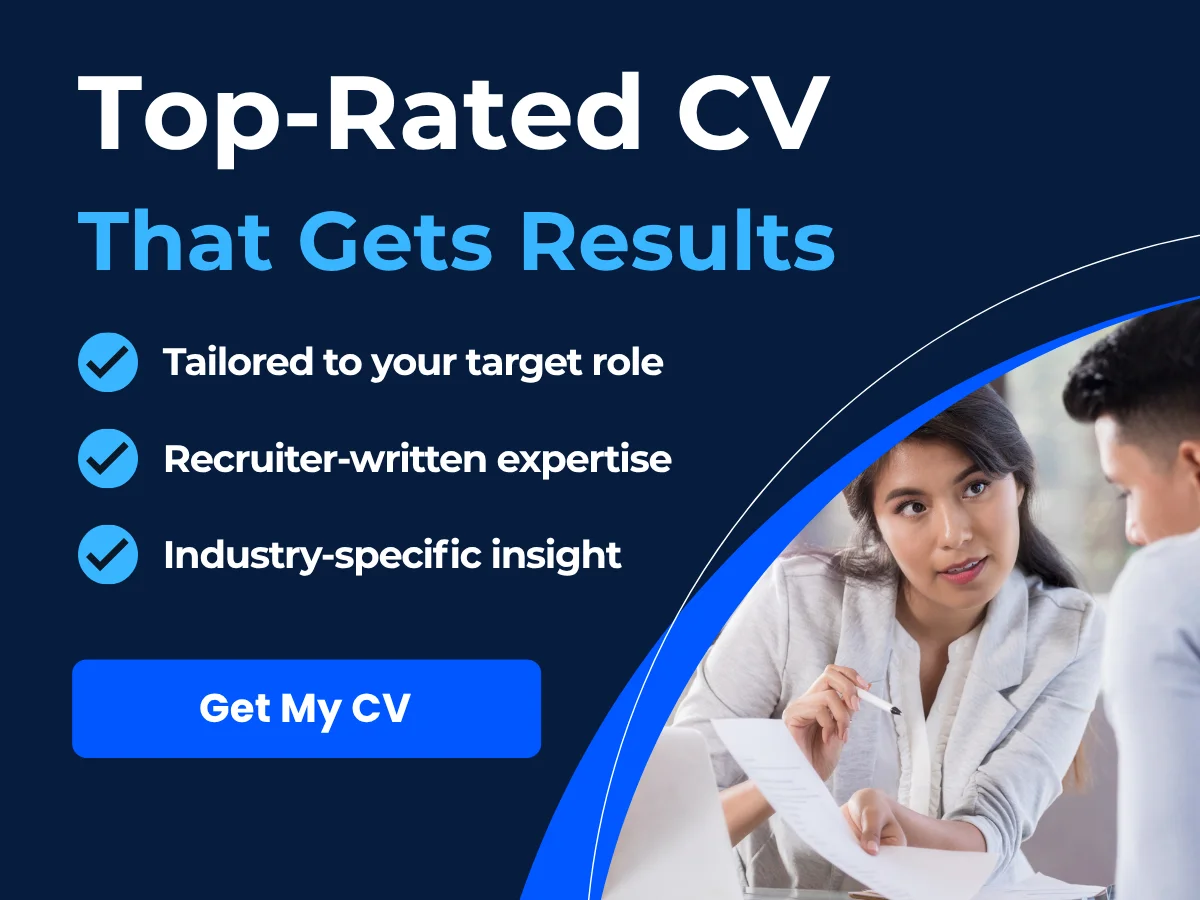
Types of Data Structures
Data structures can be broadly categorized into two main types: linear data structures and non-linear data structures. Each type has its own characteristics, advantages, and use cases.
Linear Data Structures
Linear data structures are those in which data elements are arranged in a sequential manner. Each element is connected to its previous and next element, forming a linear sequence. Common examples of linear data structures include:
- Arrays: An array is a collection of elements identified by index or key. Arrays are fixed in size and allow for fast access to elements using their index. For example, an array of integers can be declared as follows:
int[] numbers = {1, 2, 3, 4, 5};
class Node {
int data;
Node next;
}
Stack stack = new Stack<>();
Queue queue = new LinkedList<>();
Non-Linear Data Structures
Non-linear data structures are those in which data elements are not arranged sequentially. Instead, they are organized in a hierarchical or interconnected manner. This allows for more complex relationships between data elements. Common examples of non-linear data structures include:
- Trees: A tree is a hierarchical structure consisting of nodes, where each node has a value and references to child nodes. The top node is called the root, and nodes without children are called leaves. Trees are widely used in various applications, such as representing hierarchical data (e.g., file systems). A binary tree, where each node has at most two children, can be represented as:
class TreeNode {
int value;
TreeNode left;
TreeNode right;
}
Map> graph = new HashMap<>();
Map hashTable = new HashMap<>();
Why are Data Structures Important?
Data structures are fundamental to computer science and programming for several reasons:
- Efficiency: The choice of data structure can greatly affect the efficiency of algorithms. For instance, searching for an element in an array has a time complexity of O(n), while searching in a hash table can be O(1) on average. Understanding data structures allows developers to choose the most efficient way to store and manipulate data.
- Memory Management: Different data structures have different memory requirements. For example, arrays require contiguous memory allocation, while linked lists can utilize non-contiguous memory. Choosing the right data structure can help optimize memory usage, especially in resource-constrained environments.
- Data Organization: Data structures provide a way to organize data in a manner that makes it easier to access and manipulate. For example, trees are ideal for representing hierarchical data, while graphs are suitable for representing relationships between entities.
- Algorithm Implementation: Many algorithms are designed to work with specific data structures. For example, depth-first search (DFS) and breadth-first search (BFS) algorithms are typically implemented using stacks and queues, respectively. Understanding data structures is crucial for implementing these algorithms effectively.
- Problem Solving: Knowledge of data structures enhances problem-solving skills. Many coding interview questions and competitive programming challenges require a solid understanding of data structures to devise efficient solutions. Familiarity with various data structures allows developers to approach problems from different angles and choose the best solution.
Data structures are a cornerstone of computer science, providing the foundation for efficient data management and manipulation. A strong grasp of data structures is essential for any aspiring software developer or computer scientist, as it directly impacts the performance and scalability of applications.
Array-Based Questions
What is an Array?
An array is a data structure that stores a fixed-size sequential collection of elements of the same type. The elements in an array are stored in contiguous memory locations, which allows for efficient access and manipulation. Arrays can be one-dimensional (like a list) or multi-dimensional (like a matrix). The primary characteristic of an array is that it allows for random access to its elements, meaning that you can access any element directly if you know its index.
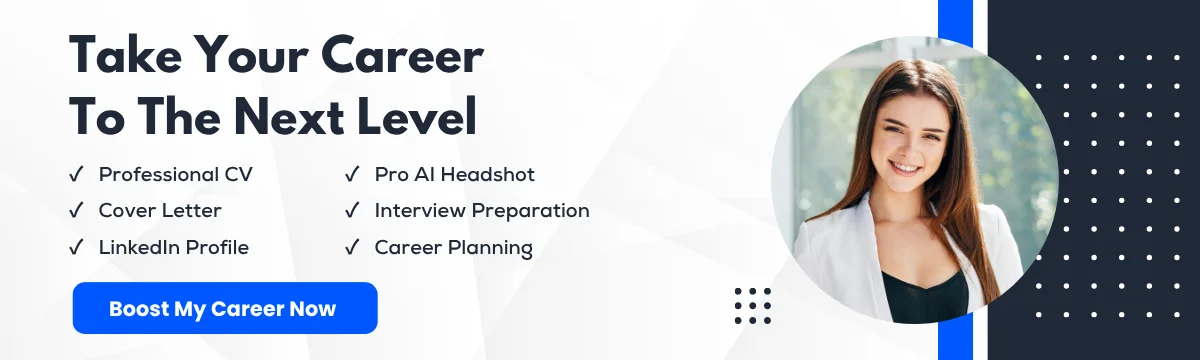
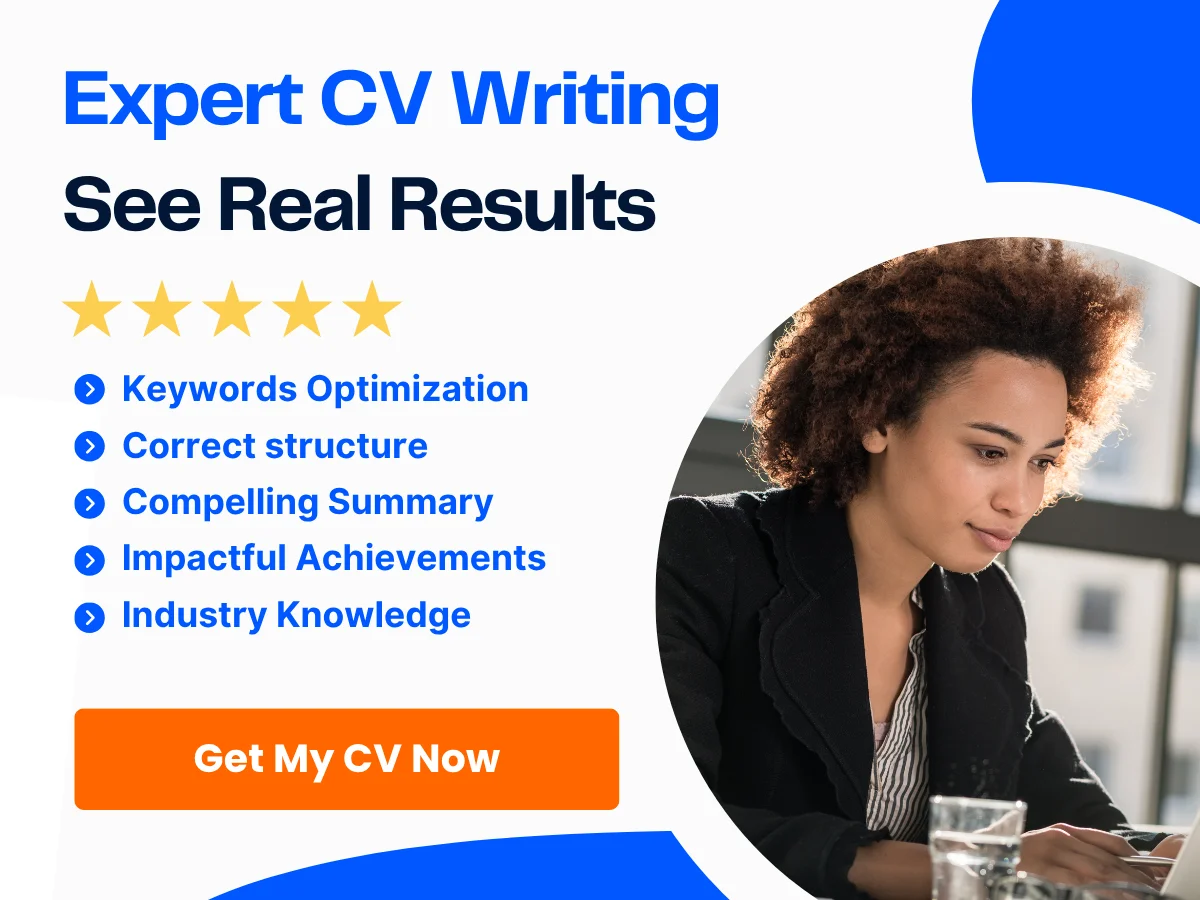
For example, consider an array of integers:
int numbers[] = {1, 2, 3, 4, 5};
In this case, the array numbers
contains five elements, and you can access the first element using numbers[0]
, the second element using numbers[1]
, and so on.
Advantages and Disadvantages of Arrays
Arrays come with their own set of advantages and disadvantages:
Advantages:
- Fast Access: Since arrays allow random access, retrieving an element by its index is a constant time operation, O(1).
- Memory Efficiency: Arrays are stored in contiguous memory locations, which can lead to better cache performance and lower memory overhead.
- Simplicity: The structure of arrays is straightforward, making them easy to implement and use.
Disadvantages:
- Fixed Size: Once an array is created, its size cannot be changed. This can lead to wasted memory if the array is too large or insufficient space if it is too small.
- Costly Insertions/Deletions: Inserting or deleting elements from an array can be expensive, as it may require shifting elements to maintain order, resulting in O(n) time complexity.
- Homogeneous Elements: Arrays can only store elements of the same type, which can limit their flexibility.
Common Operations on Arrays
Understanding how to perform common operations on arrays is crucial for any programmer. Here are some of the most common operations:
Insertion
Insertion in an array involves adding a new element at a specified index. If the array is full, you may need to create a new array with a larger size and copy the elements over. The time complexity for insertion can vary:
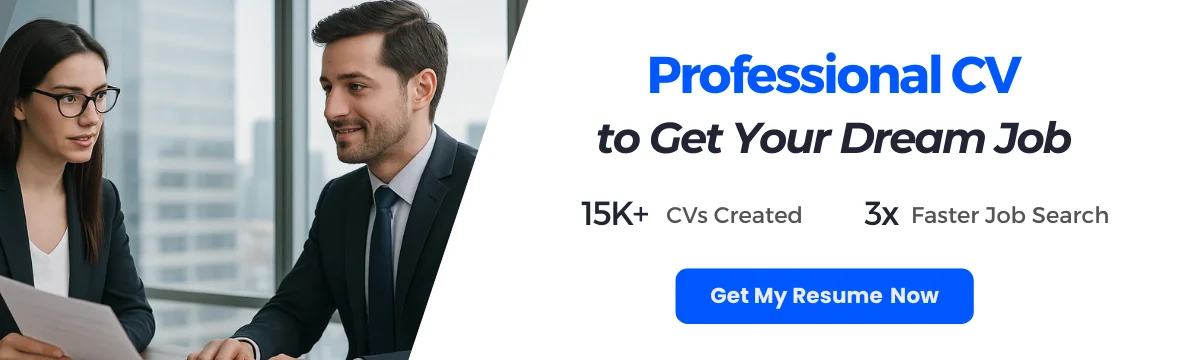
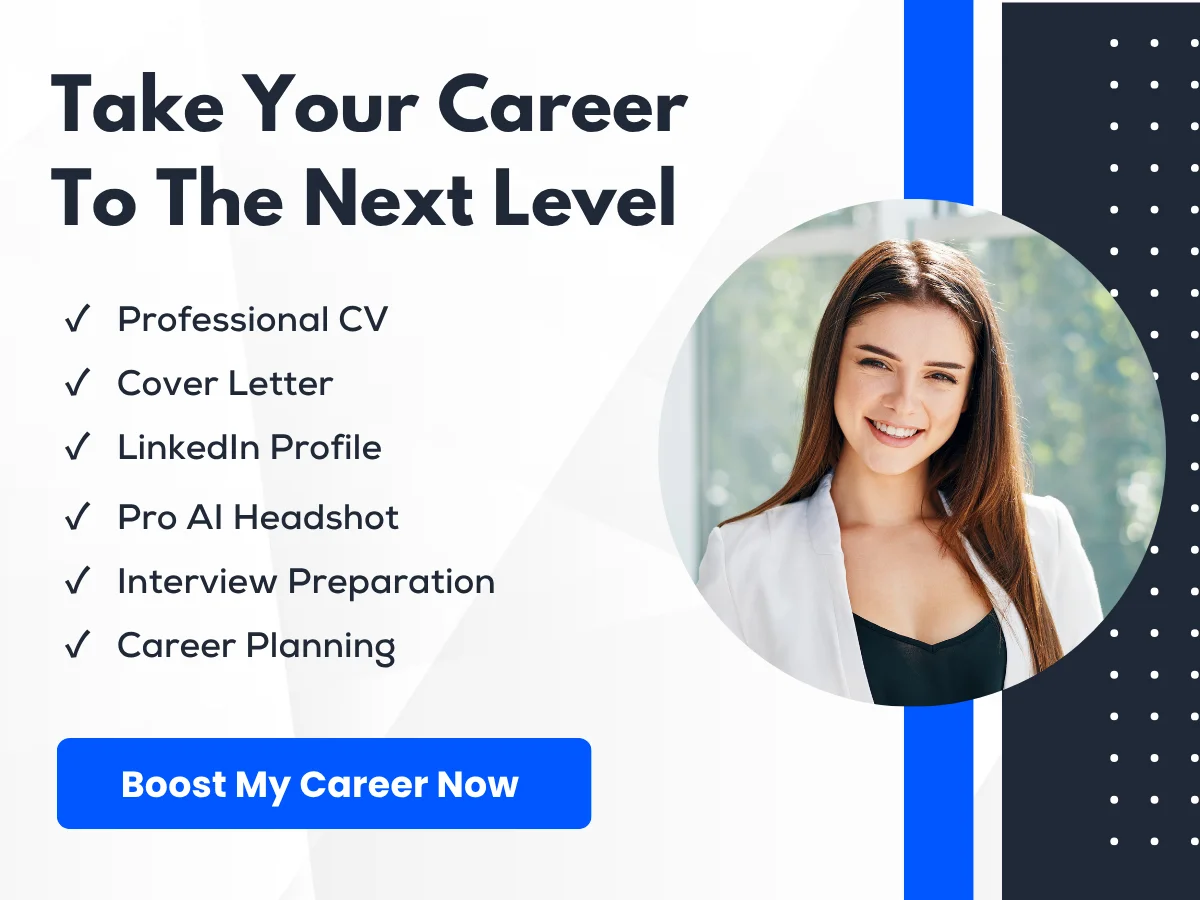
- O(n) if you need to shift elements to make space.
- O(1) if you are inserting at the end of a dynamic array (like an ArrayList in Java).
Deletion
Deletion involves removing an element from a specified index. Similar to insertion, if you delete an element, you may need to shift the remaining elements to fill the gap. The time complexity for deletion is:
- O(n) for shifting elements.
Traversal
Traversal is the process of accessing each element in the array, typically done using a loop. The time complexity for traversal is O(n), as you need to visit each element once.
Sample Interview Questions on Arrays
Here are some common interview questions related to arrays, along with explanations and sample solutions:
How to find the largest/smallest element in an array?
To find the largest or smallest element in an array, you can iterate through the array while keeping track of the maximum or minimum value found so far. Here’s a simple implementation in Python:
def find_largest(arr):
largest = arr[0]
for num in arr:
if num > largest:
largest = num
return largest
numbers = [3, 1, 4, 1, 5, 9, 2]
print(find_largest(numbers)) # Output: 9
How to reverse an array?
Reversing an array can be done in place by swapping elements from the start and end of the array until you reach the middle. Here’s how you can do it:
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
numbers = [1, 2, 3, 4, 5]
print(reverse_array(numbers)) # Output: [5, 4, 3, 2, 1]
How to remove duplicates from an array?
Removing duplicates from an array can be achieved using a set to track seen elements. Here’s a simple implementation:
def remove_duplicates(arr):
seen = set()
result = []
for num in arr:
if num not in seen:
seen.add(num)
result.append(num)
return result
numbers = [1, 2, 2, 3, 4, 4, 5]
print(remove_duplicates(numbers)) # Output: [1, 2, 3, 4, 5]
Alternatively, if you are using a language that supports it, you can use built-in functions to achieve the same result more succinctly:

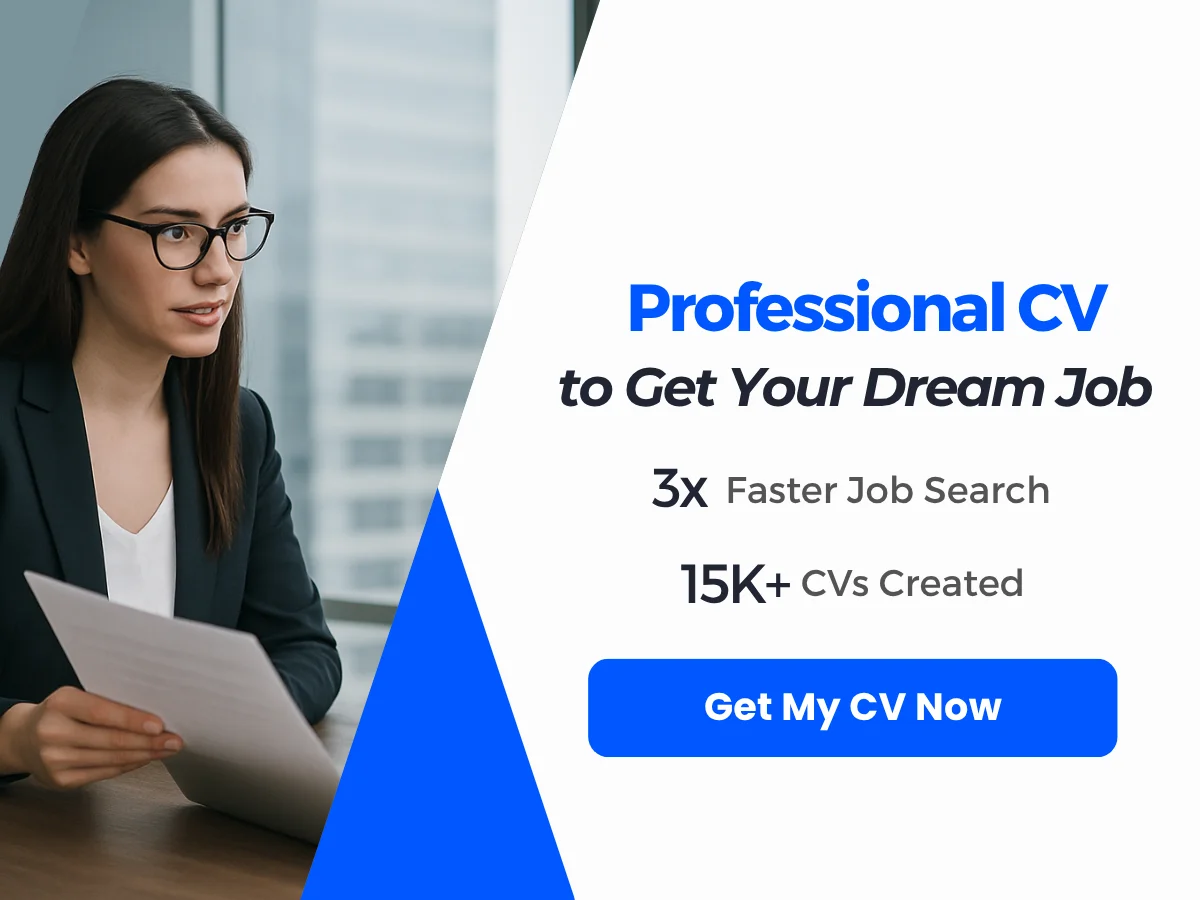
def remove_duplicates(arr):
return list(set(arr))
numbers = [1, 2, 2, 3, 4, 4, 5]
print(remove_duplicates(numbers)) # Output: [1, 2, 3, 4, 5]
Arrays are a fundamental data structure that every programmer should understand. Mastering array operations and common interview questions can significantly enhance your problem-solving skills and prepare you for technical interviews.
Linked List Questions
What is a Linked List?
A linked list is a linear data structure that consists of a sequence of elements, where each element, known as a node, contains a data field and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory allocation, which allows for efficient insertion and deletion of elements. This flexibility makes linked lists a popular choice for implementing dynamic data structures.
Each node in a linked list typically contains two components:
- Data: The value or information stored in the node.
- Next: A pointer or reference to the next node in the list.
Linked lists can grow and shrink in size dynamically, making them more versatile than arrays, which have a fixed size. However, this flexibility comes at the cost of increased memory usage due to the storage of pointers and potentially slower access times, as elements must be accessed sequentially.
Types of Linked Lists
There are several types of linked lists, each with its own characteristics and use cases:
Singly Linked List
A singly linked list is the simplest form of a linked list, where each node contains a single link to the next node. The last node points to null
, indicating the end of the list. This structure allows for efficient insertion and deletion at the beginning and end of the list, but traversal can only occur in one direction.
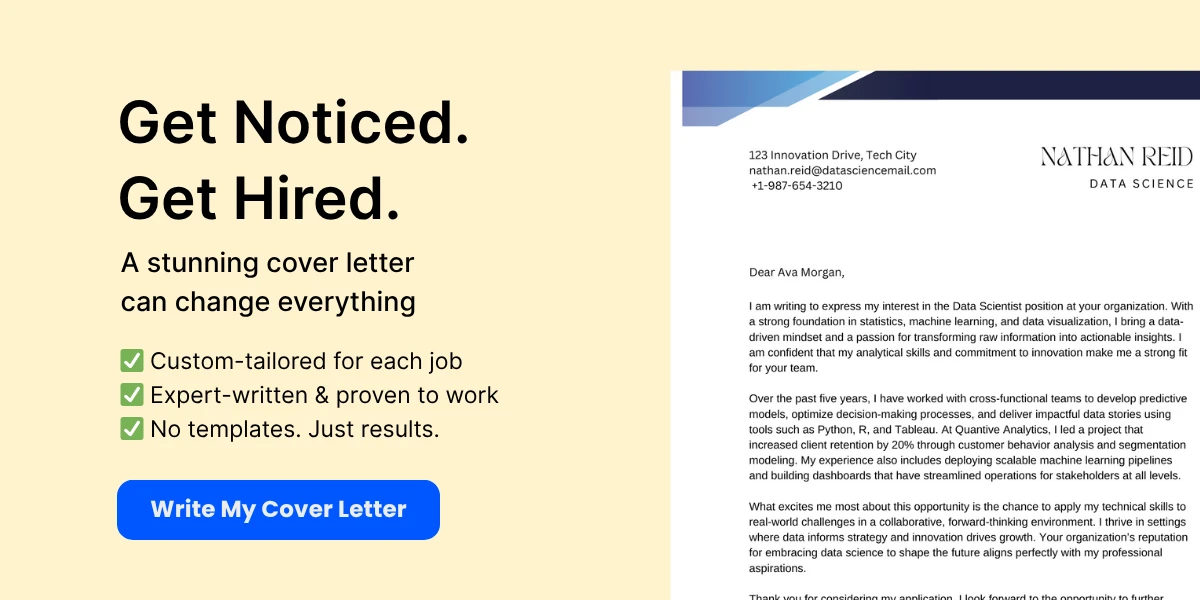
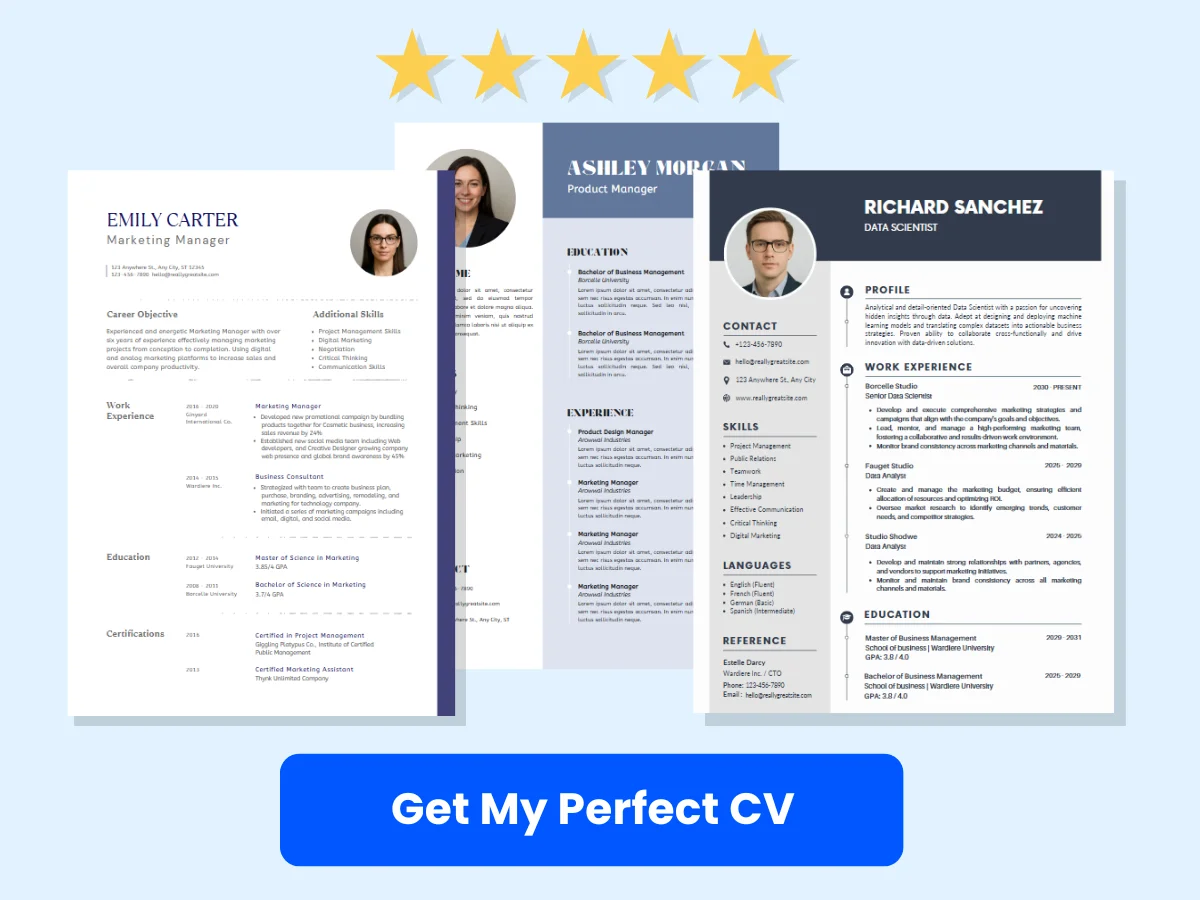
class Node {
int data;
Node next;
}
class SinglyLinkedList {
Node head;
}
Doubly Linked List
A doubly linked list extends the concept of a singly linked list by allowing each node to contain two links: one to the next node and another to the previous node. This bidirectional linking enables traversal in both directions, making operations like insertion and deletion more flexible.
class Node {
int data;
Node next;
Node prev;
}
class DoublyLinkedList {
Node head;
}
Circular Linked List
A circular linked list can be either singly or doubly linked, but with a key difference: the last node points back to the first node, creating a circular structure. This allows for continuous traversal of the list without encountering a null reference. Circular linked lists are particularly useful in applications that require a circular iteration over the elements.
class Node {
int data;
Node next;
}
class CircularLinkedList {
Node head;
}
Common Operations on Linked Lists
Linked lists support several fundamental operations that are essential for manipulating the data structure:
Insertion
Insertion in a linked list can occur at various positions:
- At the beginning: A new node is created and its
next
pointer is set to the current head. The head is then updated to point to the new node. - At the end: A new node is created, and the current last node's
next
pointer is updated to point to the new node. The new node'snext
pointer is set tonull
. - At a specific position: The list is traversed to the desired position, and the new node's
next
pointer is set to the current node at that position, while the previous node'snext
pointer is updated to point to the new node.
Deletion
Deletion operations can also occur at various positions:
- From the beginning: The head is updated to point to the second node, effectively removing the first node from the list.
- From the end: The list is traversed to find the second-to-last node, which is then updated to point to
null
. - From a specific position: The list is traversed to the node before the one to be deleted, and its
next
pointer is updated to skip the node to be deleted.
Traversal
Traversal of a linked list involves visiting each node in the list, typically starting from the head and following the next
pointers until reaching the end. This operation is essential for searching for elements, displaying the list, or performing other operations.
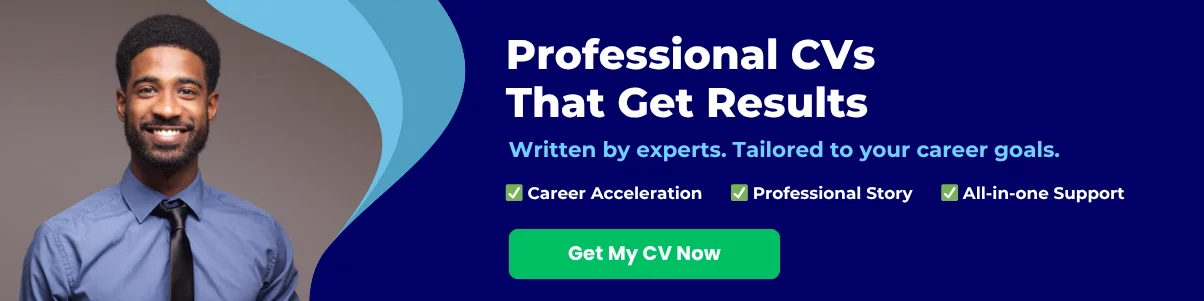
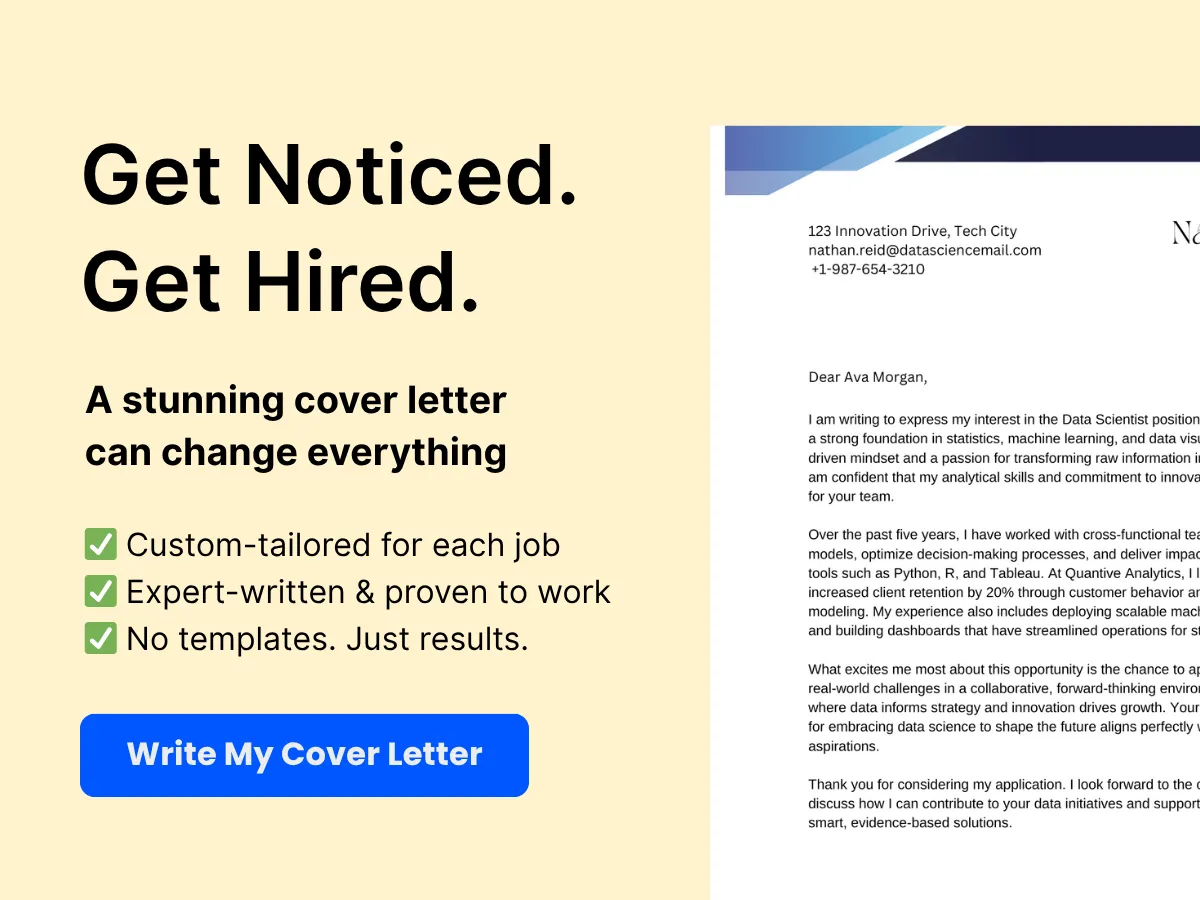
void traverse(SinglyLinkedList list) {
Node current = list.head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
}
Sample Interview Questions on Linked Lists
How to detect a cycle in a linked list?
Detecting a cycle in a linked list can be efficiently accomplished using Floyd’s Cycle-Finding Algorithm, also known as the Tortoise and Hare algorithm. This method uses two pointers that traverse the list at different speeds. If there is a cycle, the fast pointer will eventually meet the slow pointer.
boolean hasCycle(Node head) {
Node slow = head;
Node fast = head;
while (fast != null && fast.next != null) {
slow = slow.next;
fast = fast.next.next;
if (slow == fast) {
return true; // Cycle detected
}
}
return false; // No cycle
}
How to reverse a linked list?
Reversing a linked list involves changing the direction of the next
pointers so that they point to the previous node instead of the next one. This can be done iteratively or recursively. The iterative approach is more commonly used due to its simplicity and efficiency.
Node reverse(Node head) {
Node prev = null;
Node current = head;
while (current != null) {
Node next = current.next; // Store next node
current.next = prev; // Reverse the link
prev = current; // Move prev and current one step forward
current = next;
}
return prev; // New head of the reversed list
}
How to merge two sorted linked lists?
Merging two sorted linked lists involves creating a new linked list that contains all the elements from both lists in sorted order. This can be achieved by comparing the head nodes of both lists and appending the smaller node to the new list, then moving the pointer of the list from which the node was taken.
Node merge(Node l1, Node l2) {
Node dummy = new Node(0); // Dummy node to simplify the merge process
Node tail = dummy;
while (l1 != null && l2 != null) {
if (l1.data < l2.data) {
tail.next = l1;
l1 = l1.next;
} else {
tail.next = l2;
l2 = l2.next;
}
tail = tail.next; // Move the tail pointer
}
tail.next = (l1 != null) ? l1 : l2; // Append the remaining nodes
return dummy.next; // Return the merged list, skipping the dummy node
}
Stack Questions
What is a Stack?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. Stacks are often visualized as a collection of items stacked on top of each other, similar to a stack of plates. The primary operations associated with stacks are push, pop, and peek.
Stacks can be implemented using arrays or linked lists, and they are widely used in various applications, including function call management in programming languages, expression evaluation, and backtracking algorithms.
Stack Operations
Push
The push operation adds an element to the top of the stack. When an element is pushed onto the stack, it becomes the new top element. If the stack is implemented using an array, the push operation involves incrementing the top index and placing the new element at that index. If the stack is implemented using a linked list, a new node is created and linked to the current top node.
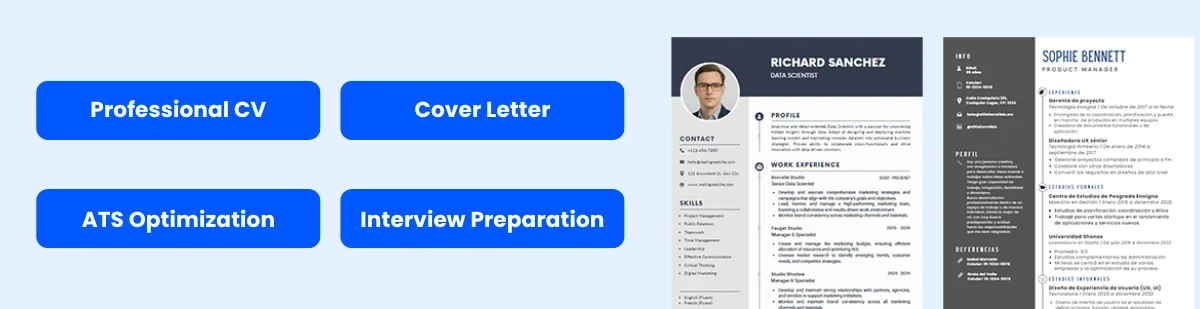
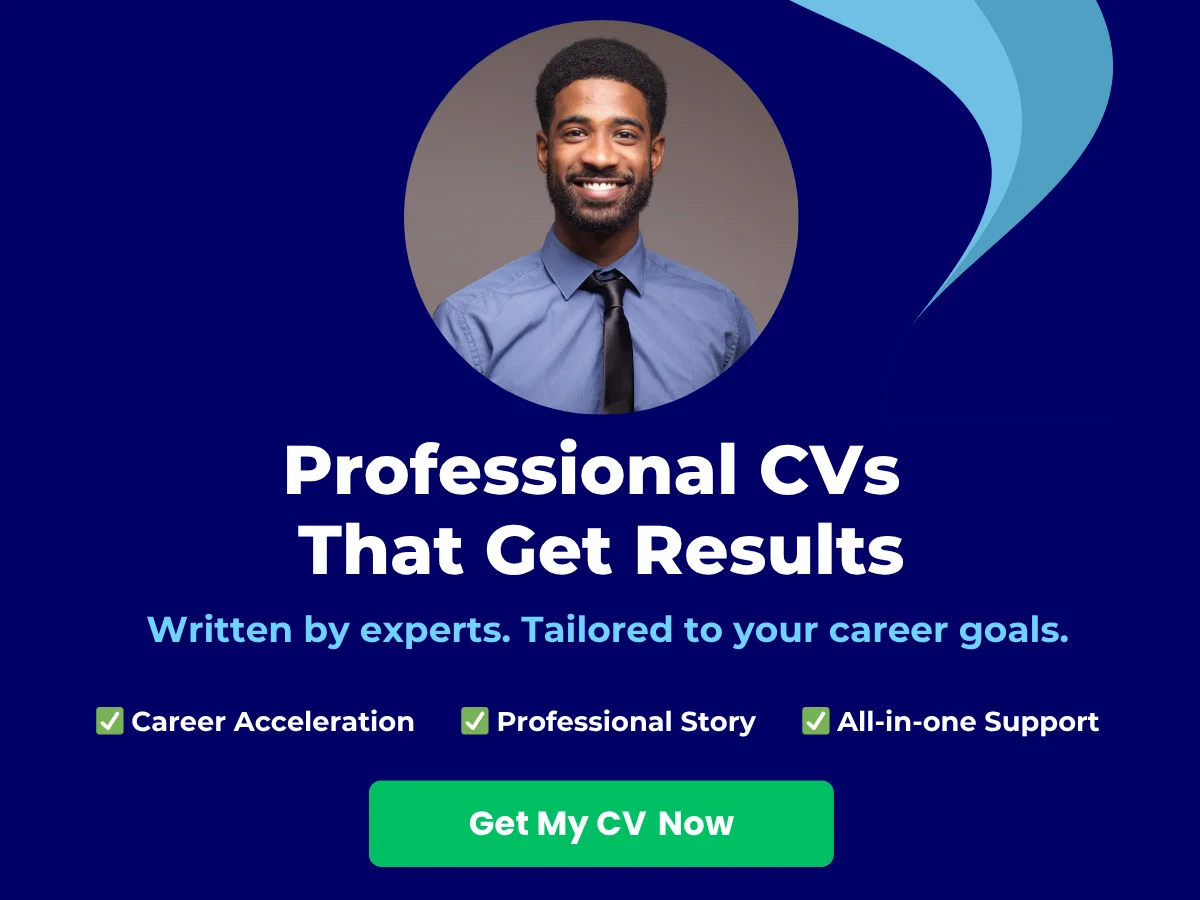
function push(stack, element) {
stack[++stack.top] = element; // For array implementation
}
Pop
The pop operation removes the top element from the stack and returns it. If the stack is empty, a pop operation may result in an underflow condition. In an array implementation, the top index is decremented, and the element at that index is returned. In a linked list implementation, the current top node is removed, and the next node becomes the new top.
function pop(stack) {
if (stack.top == -1) {
throw new Error("Stack Underflow");
}
return stack[stack.top--]; // For array implementation
}
Peek
The peek operation retrieves the top element of the stack without removing it. This operation is useful when you want to see what the last added element is without modifying the stack's state. In both array and linked list implementations, peek simply returns the value of the top element.
function peek(stack) {
if (stack.top == -1) {
throw new Error("Stack is empty");
}
return stack[stack.top]; // For array implementation
}
Applications of Stacks
Stacks have numerous applications in computer science and programming. Some of the most common applications include:
- Function Call Management: Stacks are used to manage function calls in programming languages. When a function is called, its execution context is pushed onto the stack, and when the function returns, the context is popped off.
- Expression Evaluation: Stacks are used to evaluate expressions, especially in postfix notation (Reverse Polish Notation). They help in converting infix expressions to postfix and evaluating them efficiently.
- Backtracking Algorithms: Stacks are used in algorithms that require backtracking, such as solving mazes or puzzles. They help keep track of the previous states and allow the algorithm to revert to them when necessary.
- Undo Mechanism: Many applications, such as text editors, use stacks to implement the undo feature. Each action is pushed onto a stack, and when the user wants to undo an action, the last action is popped from the stack.
Sample Interview Questions on Stacks
How to implement a stack using arrays?
To implement a stack using arrays, you need to maintain an array to hold the stack elements and an integer to track the index of the top element. Here’s a simple implementation in JavaScript:
class Stack {
constructor(size) {
this.stack = new Array(size);
this.top = -1; // Indicates an empty stack
}
push(element) {
if (this.top === this.stack.length - 1) {
throw new Error("Stack Overflow");
}
this.stack[++this.top] = element;
}
pop() {
if (this.top === -1) {
throw new Error("Stack Underflow");
}
return this.stack[this.top--];
}
peek() {
if (this.top === -1) {
throw new Error("Stack is empty");
}
return this.stack[this.top];
}
isEmpty() {
return this.top === -1;
}
}
How to implement a stack using linked lists?
To implement a stack using linked lists, you need to create a node structure that contains the data and a pointer to the next node. The top of the stack will be represented by the head of the linked list. Here’s a simple implementation in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Stack:
def __init__(self):
self.top = None
def push(self, data):
new_node = Node(data)
new_node.next = self.top
self.top = new_node
def pop(self):
if self.is_empty():
raise Exception("Stack Underflow")
popped_node = self.top
self.top = self.top.next
return popped_node.data
def peek(self):
if self.is_empty():
raise Exception("Stack is empty")
return self.top.data
def is_empty(self):
return self.top is None
How to evaluate a postfix expression using a stack?
To evaluate a postfix expression, you can use a stack to store operands. When an operator is encountered, the required number of operands is popped from the stack, the operation is performed, and the result is pushed back onto the stack. Here’s a simple implementation in Python:
def evaluate_postfix(expression):
stack = []
for char in expression.split():
if char.isdigit(): # If the character is an operand
stack.append(int(char))
else: # The character is an operator
operand2 = stack.pop()
operand1 = stack.pop()
if char == '+':
stack.append(operand1 + operand2)
elif char == '-':
stack.append(operand1 - operand2)
elif char == '*':
stack.append(operand1 * operand2)
elif char == '/':
stack.append(operand1 / operand2)
return stack.pop()
# Example usage
postfix_expression = "3 4 + 2 * 7 /"
result = evaluate_postfix(postfix_expression)
print("Result:", result) # Output: Result: 2.0
In this example, the postfix expression "3 4 + 2 * 7 /" is evaluated step by step using a stack, resulting in the final output.
Queue Questions
What is a Queue?
A queue is a linear data structure that follows the First In First Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed. Queues are commonly used in scenarios where order needs to be preserved, such as in scheduling tasks, managing requests in a server, or handling asynchronous data transfers.
In a queue, elements are added at one end, known as the rear, and removed from the other end, known as the front. This structure is analogous to a line of people waiting for service, where the first person in line is the first to be served.
Types of Queues
Queues can be categorized into several types based on their structure and functionality:
Simple Queue
A simple queue is the most basic type of queue. It allows insertion of elements at the rear and deletion from the front. The operations are straightforward, but it can lead to inefficient use of space if elements are removed from the front and new elements are added to the rear, as the front may become empty while the rear is filled.
Circular Queue
A circular queue addresses the limitations of a simple queue by connecting the end of the queue back to the front, forming a circle. This allows for efficient use of space, as once the rear reaches the end of the array, it can wrap around to the beginning if there is space available. The circular queue maintains the FIFO order while optimizing memory usage.
Priority Queue
A priority queue is a special type of queue where each element is associated with a priority. Elements with higher priority are dequeued before those with lower priority, regardless of their order in the queue. This is particularly useful in scenarios like task scheduling, where certain tasks must be completed before others, regardless of their arrival time.
Deque (Double-Ended Queue)
A deque (pronounced "deck") is a double-ended queue that allows insertion and deletion of elements from both the front and the rear. This flexibility makes deques suitable for a variety of applications, such as implementing stacks, queues, and even certain algorithms that require access to both ends of the data structure.
Queue Operations
Queues support several fundamental operations that allow for the management of elements:
Enqueue
The enqueue operation adds an element to the rear of the queue. In a simple queue, this operation is straightforward, but in a circular queue, care must be taken to ensure that the rear wraps around correctly if it reaches the end of the underlying array.
function enqueue(queue, element) {
if (isFull(queue)) {
throw new Error("Queue is full");
}
queue.rear = (queue.rear + 1) % queue.size;
queue.elements[queue.rear] = element;
}
Dequeue
The dequeue operation removes an element from the front of the queue. This operation is also straightforward in a simple queue, but in a circular queue, the front index must be updated correctly to maintain the circular nature.
function dequeue(queue) {
if (isEmpty(queue)) {
throw new Error("Queue is empty");
}
const element = queue.elements[queue.front];
queue.front = (queue.front + 1) % queue.size;
return element;
}
Front
The front operation retrieves the element at the front of the queue without removing it. This is useful for checking which element will be dequeued next.
function front(queue) {
if (isEmpty(queue)) {
throw new Error("Queue is empty");
}
return queue.elements[queue.front];
}
Rear
The rear operation retrieves the element at the rear of the queue without removing it. This can be useful for checking the last element added to the queue.
function rear(queue) {
if (isEmpty(queue)) {
throw new Error("Queue is empty");
}
return queue.elements[queue.rear];
}
Sample Interview Questions on Queues
Understanding queues is crucial for technical interviews, especially for roles involving data structures and algorithms. Here are some common interview questions related to queues:
How to implement a queue using arrays?
To implement a queue using arrays, you can create a fixed-size array and maintain two pointers: one for the front and one for the rear. The enqueue operation adds an element at the rear, while the dequeue operation removes an element from the front. Care must be taken to handle the case when the queue is full or empty.
class Queue {
constructor(size) {
this.size = size;
this.elements = new Array(size);
this.front = 0;
this.rear = -1;
this.count = 0;
}
enqueue(element) {
if (this.count === this.size) {
throw new Error("Queue is full");
}
this.rear = (this.rear + 1) % this.size;
this.elements[this.rear] = element;
this.count++;
}
dequeue() {
if (this.count === 0) {
throw new Error("Queue is empty");
}
const element = this.elements[this.front];
this.front = (this.front + 1) % this.size;
this.count--;
return element;
}
}
How to implement a queue using linked lists?
Implementing a queue using linked lists involves creating a linked list where each node contains a data element and a pointer to the next node. The front of the queue corresponds to the head of the linked list, while the rear corresponds to the tail. This implementation allows for dynamic sizing, as elements can be added or removed without worrying about a fixed size.
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class LinkedListQueue {
constructor() {
this.front = null;
this.rear = null;
}
enqueue(data) {
const newNode = new Node(data);
if (this.rear) {
this.rear.next = newNode;
}
this.rear = newNode;
if (!this.front) {
this.front = newNode;
}
}
dequeue() {
if (!this.front) {
throw new Error("Queue is empty");
}
const data = this.front.data;
this.front = this.front.next;
if (!this.front) {
this.rear = null;
}
return data;
}
}
How to implement a circular queue?
To implement a circular queue, you can use a fixed-size array and maintain two pointers (front and rear) along with a count of the number of elements. The key difference from a simple queue is that when the rear reaches the end of the array, it wraps around to the beginning if there is space available. This implementation ensures that the queue can utilize the array space efficiently.
class CircularQueue {
constructor(size) {
this.size = size;
this.elements = new Array(size);
this.front = 0;
this.rear = -1;
this.count = 0;
}
enqueue(element) {
if (this.count === this.size) {
throw new Error("Queue is full");
}
this.rear = (this.rear + 1) % this.size;
this.elements[this.rear] = element;
this.count++;
}
dequeue() {
if (this.count === 0) {
throw new Error("Queue is empty");
}
const element = this.elements[this.front];
this.front = (this.front + 1) % this.size;
this.count--;
return element;
}
}
Tree Questions
What is a Tree?
A tree is a widely used abstract data type that simulates a hierarchical structure. It consists of nodes connected by edges, where each tree has a single root node and zero or more child nodes. The root node is the topmost node in the tree, and every other node can be reached from the root by traversing down the tree. Trees are used in various applications, including databases, file systems, and network routing algorithms.
In a tree, each node contains a value or data, and it may also have links to other nodes (its children). The nodes without children are called leaf nodes. The depth of a node is defined as the number of edges from the root to that node, while the height of a tree is the maximum depth among all its nodes.
Types of Trees
Binary Tree
A binary tree is a type of tree where each node has at most two children, referred to as the left child and the right child. This structure allows for efficient searching, insertion, and deletion operations. The binary tree can be further classified into various types based on the arrangement of nodes.
Binary Search Tree (BST)
A binary search tree is a special type of binary tree that maintains a sorted order of its elements. In a BST, for any given node:
- All values in the left subtree are less than the node's value.
- All values in the right subtree are greater than the node's value.
This property allows for efficient searching, insertion, and deletion operations, typically with a time complexity of O(log n) for balanced trees.
AVL Tree
An AVL tree is a self-balancing binary search tree where the difference in heights between the left and right subtrees (the balance factor) is at most one for every node. This balancing ensures that the tree remains approximately balanced, leading to O(log n) time complexity for search, insertion, and deletion operations. AVL trees require rotations to maintain balance after insertions and deletions.
Red-Black Tree
A red-black tree is another type of self-balancing binary search tree. Each node in a red-black tree has an additional bit for denoting the color of the node, either red or black. The tree must satisfy the following properties:
- The root is always black.
- Red nodes cannot have red children (no two red nodes can be adjacent).
- Every path from a node to its descendant leaf nodes must have the same number of black nodes.
These properties ensure that the tree remains balanced, allowing for O(log n) time complexity for search, insertion, and deletion operations.
B-Tree
A B-tree is a self-balancing tree data structure that maintains sorted data and allows searches, sequential access, insertions, and deletions in logarithmic time. B-trees are commonly used in databases and file systems. Unlike binary trees, B-trees can have more than two children per node, which helps to minimize the number of disk accesses required for large datasets. The order of a B-tree defines the maximum number of children each node can have.
Tree Traversal Techniques
Tree traversal refers to the process of visiting all the nodes in a tree data structure in a specific order. There are several methods for traversing trees, each with its own use cases.
In-order
In-order traversal visits the nodes of a binary tree in the following order: left subtree, root node, right subtree. This traversal method is particularly useful for binary search trees, as it retrieves the values in sorted order.
function inOrderTraversal(node) {
if (node != null) {
inOrderTraversal(node.left);
console.log(node.value);
inOrderTraversal(node.right);
}
}
Pre-order
Pre-order traversal visits the nodes in the following order: root node, left subtree, right subtree. This method is useful for creating a copy of the tree or for prefix expression evaluation.
function preOrderTraversal(node) {
if (node != null) {
console.log(node.value);
preOrderTraversal(node.left);
preOrderTraversal(node.right);
}
}
Post-order
Post-order traversal visits the nodes in the following order: left subtree, right subtree, root node. This method is useful for deleting a tree or for postfix expression evaluation.
function postOrderTraversal(node) {
if (node != null) {
postOrderTraversal(node.left);
postOrderTraversal(node.right);
console.log(node.value);
}
}
Level-order
Level-order traversal visits the nodes level by level, starting from the root and moving down to each subsequent level. This method is often implemented using a queue and is useful for finding the shortest path in unweighted trees.
function levelOrderTraversal(root) {
if (root == null) return;
let queue = [];
queue.push(root);
while (queue.length > 0) {
let node = queue.shift();
console.log(node.value);
if (node.left != null) queue.push(node.left);
if (node.right != null) queue.push(node.right);
}
}
Sample Interview Questions on Trees
How to find the height of a binary tree?
The height of a binary tree is defined as the number of edges on the longest path from the root to a leaf node. To find the height, we can use a recursive approach:
function findHeight(node) {
if (node == null) return -1; // height of an empty tree is -1
return Math.max(findHeight(node.left), findHeight(node.right)) + 1;
}
How to check if a binary tree is a BST?
To determine if a binary tree is a binary search tree, we can perform an in-order traversal and check if the values are in sorted order. Alternatively, we can use a recursive approach that checks if each node's value falls within a valid range:
function isBST(node, min = null, max = null) {
if (node == null) return true;
if ((min != null && node.value <= min) || (max != null && node.value >= max)) {
return false;
}
return isBST(node.left, min, node.value) && isBST(node.right, node.value, max);
}
How to find the lowest common ancestor (LCA) in a binary tree?
The lowest common ancestor of two nodes in a binary tree is defined as the deepest node that is an ancestor of both nodes. To find the LCA, we can use a recursive approach:
function findLCA(root, n1, n2) {
if (root == null) return null;
if (root.value === n1 || root.value === n2) return root;
let leftLCA = findLCA(root.left, n1, n2);
let rightLCA = findLCA(root.right, n1, n2);
if (leftLCA && rightLCA) return root;
return leftLCA != null ? leftLCA : rightLCA;
}
Graph Questions
What is a Graph?
A graph is a fundamental data structure used to represent relationships between pairs of objects. It consists of a set of vertices (or nodes) and a set of edges that connect these vertices. Graphs are widely used in various applications, including social networks, transportation systems, and web page linking. The versatility of graphs allows them to model complex relationships and interactions in a structured way.
Types of Graphs
Graphs can be classified into several types based on their properties and the nature of their edges. Understanding these types is crucial for solving graph-related problems effectively.
Directed Graph
A directed graph, or digraph, is a graph where the edges have a direction. This means that each edge connects an ordered pair of vertices, indicating a one-way relationship. For example, in a social network, if person A follows person B, this relationship can be represented as a directed edge from A to B.
Undirected Graph
In contrast, an undirected graph has edges that do not have a direction. The relationship between the vertices is bidirectional. For instance, if two people are friends, the relationship can be represented as an undirected edge between them, indicating that both individuals are connected to each other.
Weighted Graph
A weighted graph assigns a weight or cost to each edge, representing the cost of traversing that edge. This is particularly useful in scenarios like finding the shortest path in a network, where the weights could represent distances, costs, or time. For example, in a road network, the weight of an edge could represent the distance between two cities.
Unweighted Graph
An unweighted graph does not assign any weights to its edges. All edges are considered equal, which simplifies many graph algorithms. Unweighted graphs are often used in scenarios where the relationships are binary, such as connectivity or adjacency.
Graph Representation
Graphs can be represented in various ways, each with its advantages and disadvantages. The choice of representation can significantly affect the performance of graph algorithms.
Adjacency Matrix
An adjacency matrix is a 2D array used to represent a graph. If there are n vertices in the graph, the adjacency matrix will be an n x n matrix. The element at row i and column j indicates whether there is an edge from vertex i to vertex j. In a directed graph, this matrix will have a value of 1 if there is a directed edge from i to j, and 0 otherwise. For undirected graphs, the matrix is symmetric.
Example:
For a directed graph with vertices A, B, and C, the adjacency matrix might look like this:
A B C
A 0 1 0
B 0 0 1
C 0 0 0
Adjacency List
An adjacency list is a more space-efficient way to represent a graph. It consists of an array of lists. The index of the array represents a vertex, and each element in the list at that index represents the vertices that are adjacent to it. This representation is particularly useful for sparse graphs, where the number of edges is much less than the maximum possible number of edges.
Example:
For the same directed graph, the adjacency list would look like this:
A: B
B: C
C:
Graph Traversal Techniques
Graph traversal techniques are essential for exploring the nodes and edges of a graph. The two most common methods are Depth-First Search (DFS) and Breadth-First Search (BFS).
Depth-First Search (DFS)
DFS is a traversal technique that explores as far as possible along each branch before backtracking. It can be implemented using recursion or a stack. The algorithm starts at a selected node (the root) and explores each branch before moving to the next sibling node.
Example of DFS:
1. Start at node A
2. Visit A, then go to B
3. Visit B, then go to C
4. Since C has no unvisited adjacent nodes, backtrack to B, then to A
5. Visit the next unvisited node
Breadth-First Search (BFS)
BFS is another traversal technique that explores all the neighbor nodes at the present depth prior to moving on to nodes at the next depth level. It uses a queue to keep track of the nodes to be explored. BFS is particularly useful for finding the shortest path in unweighted graphs.
Example of BFS:
1. Start at node A
2. Visit A, then enqueue its neighbors B and C
3. Dequeue B, visit it, then enqueue its neighbors
4. Continue until all nodes are visited
Sample Interview Questions on Graphs
When preparing for interviews, it's essential to practice common graph-related questions. Here are some sample questions along with brief explanations of how to approach them.
How to detect a cycle in a graph?
To detect a cycle in a graph, you can use DFS. During the traversal, keep track of the visited nodes and the recursion stack. If you encounter a node that is already in the recursion stack, a cycle exists. For undirected graphs, you also need to ensure that you do not count the edge leading back to the parent node as a cycle.
How to find the shortest path in a weighted graph?
The Dijkstra's algorithm is commonly used to find the shortest path in a weighted graph. It maintains a priority queue of nodes to explore, starting from the source node. The algorithm updates the shortest known distance to each node and continues until all nodes have been processed. For graphs with negative weights, the Bellman-Ford algorithm is a better choice.
How to implement DFS and BFS?
Implementing DFS and BFS can be done using simple algorithms. Below are basic implementations in Python:
# DFS Implementation
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
return visited
# BFS Implementation
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
vertex = queue.popleft()
if vertex not in visited:
visited.add(vertex)
queue.extend(neighbor for neighbor in graph[vertex] if neighbor not in visited)
return visited
Understanding these concepts and practicing these questions will significantly enhance your ability to tackle graph-related problems in interviews. Mastery of graph theory is not only crucial for technical interviews but also for real-world applications in software development and data analysis.
Hashing Questions
What is Hashing?
Hashing is a process used to convert an input (or 'key') into a fixed-size string of bytes. The output, typically a hash code, is a numerical representation of the input data. Hashing is widely used in various applications, particularly in data structures like hash tables, where it allows for efficient data retrieval.
The primary purpose of hashing is to enable quick data access. By transforming data into a hash code, we can store it in a way that allows for rapid lookups, insertions, and deletions. Hashing is particularly useful in scenarios where we need to manage large datasets and require constant time complexity for these operations.
Hash Functions
A hash function is a specific algorithm that takes an input and produces a hash code. The quality of a hash function is determined by several factors:
- Deterministic: The same input should always produce the same output.
- Uniform Distribution: The hash function should distribute hash codes uniformly across the output space to minimize collisions.
- Efficient Computation: The function should be quick to compute, even for large inputs.
- Pre-image Resistance: It should be computationally infeasible to reverse the hash function, meaning you cannot easily derive the original input from the hash code.
- Collision Resistance: It should be difficult to find two different inputs that produce the same hash code.
Common examples of hash functions include MD5, SHA-1, and SHA-256. Each of these functions has its own use cases and security implications, especially in cryptographic applications.
Collision Resolution Techniques
Collisions occur when two different inputs produce the same hash code. Since hash tables rely on unique keys for efficient data retrieval, handling collisions is crucial. There are several techniques to resolve collisions:
Chaining
Chaining is a collision resolution technique where each slot in the hash table contains a linked list (or another data structure) of all entries that hash to the same index. When a collision occurs, the new entry is simply added to the list at that index.
For example, consider a hash table with a size of 10 and a simple hash function that returns the remainder of the key divided by 10. If we insert the keys 12, 22, and 32, they all hash to index 2:
Index 0: []
Index 1: []
Index 2: [12 -> 22 -> 32]
Index 3: []
...
Index 9: []
Chaining is simple to implement and can handle a large number of collisions, but it may lead to increased memory usage if many entries hash to the same index.
Open Addressing
Open addressing is another collision resolution technique where, upon a collision, the algorithm searches for the next available slot in the hash table. There are several probing methods used in open addressing:
- Linear Probing: If a collision occurs, the algorithm checks the next slot in a linear fashion until an empty slot is found.
- Quadratic Probing: Instead of checking the next slot, the algorithm checks slots at increasing intervals (1, 4, 9, etc.) to find an empty slot.
- Double Hashing: A second hash function is used to determine the step size for probing, which helps to reduce clustering.
For instance, if we have a hash table of size 10 and we insert the keys 12 and 22, both of which hash to index 2, linear probing would place the second key in index 3:
Index 0: []
Index 1: []
Index 2: [12]
Index 3: [22]
...
Index 9: []
Open addressing can be more memory efficient than chaining, but it can lead to clustering, where a group of consecutive slots are filled, making future insertions slower.
Applications of Hashing
Hashing has numerous applications across various domains:
- Data Retrieval: Hash tables provide efficient data retrieval, making them ideal for implementing associative arrays and databases.
- Cryptography: Hash functions are used in digital signatures, password storage, and data integrity checks.
- Caching: Hashing is used in caching mechanisms to quickly retrieve frequently accessed data.
- Load Balancing: Hashing can distribute requests evenly across servers in a network.
- Data Deduplication: Hashing helps identify duplicate data by comparing hash codes instead of the actual data.
Sample Interview Questions on Hashing
When preparing for interviews, it's essential to understand both the theoretical and practical aspects of hashing. Here are some common interview questions related to hashing:
How to implement a hash table?
Implementing a hash table involves creating a data structure that can store key-value pairs and handle collisions. A basic implementation in Python might look like this:
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(size)]
def hash_function(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self.hash_function(key)
for kv in self.table[index]:
if kv[0] == key:
kv[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self.hash_function(key)
for kv in self.table[index]:
if kv[0] == key:
return kv[1]
return None
def delete(self, key):
index = self.hash_function(key)
for i, kv in enumerate(self.table[index]):
if kv[0] == key:
del self.table[index][i]
return
This implementation uses chaining for collision resolution and provides methods for inserting, retrieving, and deleting key-value pairs.
How to handle collisions in a hash table?
Collisions can be handled using various techniques, as discussed earlier. The choice of technique depends on the specific requirements of the application, such as memory constraints and expected load factor. For example, if memory usage is a concern, open addressing might be preferred, while chaining could be more suitable for applications with a high number of collisions.
How to design a hash function?
Designing a good hash function involves ensuring that it meets the criteria of being deterministic, uniformly distributing keys, and being efficient to compute. A simple approach is to use the built-in hash function of the programming language and combine it with a modulus operation to fit the hash table size. For example:
def custom_hash(key, table_size):
return hash(key) % table_size
For more complex data types, such as strings or objects, you may need to combine the hash values of individual components to create a unique hash code. This can be done using techniques like polynomial rolling hash or by combining hash values using XOR operations.
Ultimately, the goal is to minimize collisions and ensure that the hash function performs well across a wide range of inputs.
Advanced Data Structure Questions
What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. In a heap, the parent node is either greater than or equal to (in a max-heap) or less than or equal to (in a min-heap) its child nodes. This property makes heaps useful for implementing priority queues, where the highest (or lowest) priority element can be accessed quickly.
Types of Heaps
Heaps can be categorized into two main types:
- Min-Heap: In a min-heap, the value of each node is less than or equal to the values of its children. This means that the smallest element is always at the root of the heap.
- Max-Heap: In a max-heap, the value of each node is greater than or equal to the values of its children. Thus, the largest element is always at the root.
Heap Operations
Heaps support several key operations that are essential for their functionality:
Insertion
To insert a new element into a heap, the element is initially added at the end of the heap (maintaining the complete binary tree property). After insertion, the heap property must be restored by "bubbling up" the new element. This involves comparing the new element with its parent and swapping them if the heap property is violated.
function insert(heap, element) {
heap.push(element);
let index = heap.length - 1;
while (index > 0) {
let parentIndex = Math.floor((index - 1) / 2);
if (heap[index] < heap[parentIndex]) {
[heap[index], heap[parentIndex]] = [heap[parentIndex], heap[index]];
index = parentIndex;
} else {
break;
}
}
}
Deletion
Deletion in a heap typically refers to removing the root element. In a min-heap, this is the smallest element, while in a max-heap, it is the largest. To delete the root, the last element in the heap is moved to the root position, and then the heap property is restored by "bubbling down" this element. This involves comparing it with its children and swapping it with the smaller (or larger) child as necessary.
function deleteRoot(heap) {
if (heap.length === 0) return null;
const root = heap[0];
heap[0] = heap.pop();
let index = 0;
while (true) {
let leftChildIndex = 2 * index + 1;
let rightChildIndex = 2 * index + 2;
let smallestIndex = index;
if (leftChildIndex < heap.length && heap[leftChildIndex] < heap[smallestIndex]) {
smallestIndex = leftChildIndex;
}
if (rightChildIndex < heap.length && heap[rightChildIndex] < heap[smallestIndex]) {
smallestIndex = rightChildIndex;
}
if (smallestIndex === index) break;
[heap[index], heap[smallestIndex]] = [heap[smallestIndex], heap[index]];
index = smallestIndex;
}
return root;
}
Heapify
The heapify operation is used to convert an arbitrary array into a heap. This can be done in two ways: top-down or bottom-up. The bottom-up approach is more efficient and involves starting from the last non-leaf node and applying the "bubbling down" process to ensure the heap property is maintained.
function heapify(array) {
const start = Math.floor((array.length - 2) / 2);
for (let i = start; i >= 0; i--) {
bubbleDown(array, i, array.length);
}
}
function bubbleDown(array, index, length) {
let smallest = index;
const leftChild = 2 * index + 1;
const rightChild = 2 * index + 2;
if (leftChild < length && array[leftChild] < array[smallest]) {
smallest = leftChild;
}
if (rightChild < length && array[rightChild] < array[smallest]) {
smallest = rightChild;
}
if (smallest !== index) {
[array[index], array[smallest]] = [array[smallest], array[index]];
bubbleDown(array, smallest, length);
}
}
Sample Interview Questions on Heaps
- Explain the difference between a min-heap and a max-heap.
- How would you implement a priority queue using a heap?
- What is the time complexity of inserting an element into a heap?
- How can heaps be used to find the k-th largest element in an array?
How to implement a heap using arrays?
Heaps can be efficiently implemented using arrays. The parent-child relationship can be easily managed using index calculations:
- The parent of a node at index
i
is located at indexMath.floor((i - 1) / 2)
. - The left child of a node at index
i
is located at index2 * i + 1
. - The right child of a node at index
i
is located at index2 * i + 2
.
This allows for efficient access and manipulation of the heap structure without the overhead of pointers, making it a space-efficient solution.
How to find the k-th largest element in an array?
To find the k-th largest element in an array, one effective method is to use a min-heap of size k. The steps are as follows:
- Initialize a min-heap.
- Iterate through the elements of the array:
- If the size of the heap is less than k, insert the current element.
- If the size of the heap is equal to k and the current element is greater than the root of the heap, replace the root with the current element and heapify.
- After processing all elements, the root of the min-heap will be the k-th largest element.
function findKthLargest(nums, k) {
const minHeap = [];
for (const num of nums) {
if (minHeap.length < k) {
minHeap.push(num);
bubbleUp(minHeap);
} else if (num > minHeap[0]) {
minHeap[0] = num;
bubbleDown(minHeap, 0);
}
}
return minHeap[0];
}
What is a Trie?
A trie, also known as a prefix tree, is a special type of tree used to store associative data structures. A common application of tries is storing a dynamic set of strings, where the keys are usually strings. Each node in a trie represents a single character of a string, and the path from the root to a node represents the prefix of the string.
Trie Operations
Tries support several key operations:
Insertion
To insert a string into a trie, start at the root and for each character in the string, check if the character node exists. If it does not exist, create a new node. Move to the next character and repeat until the entire string is inserted.
class TrieNode {
constructor() {
this.children = {};
this.isEndOfWord = false;
}
}
class Trie {
constructor() {
this.root = new TrieNode();
}
insert(word) {
let node = this.root;
for (const char of word) {
if (!node.children[char]) {
node.children[char] = new TrieNode();
}
node = node.children[char];
}
node.isEndOfWord = true;
}
}
Deletion
To delete a string from a trie, traverse the trie to find the node corresponding to the last character of the string. If the node is marked as the end of a word, unmark it. If the node has no children, remove it from its parent. This process may require backtracking to ensure that nodes are only removed if they are not part of other words.
delete(word) {
const deleteHelper = (node, word, depth) => {
if (!node) return false;
if (depth === word.length) {
if (!node.isEndOfWord) return false;
node.isEndOfWord = false;
return Object.keys(node.children).length === 0;
}
const char = word[depth];
const shouldDeleteCurrentNode = deleteHelper(node.children[char], word, depth + 1);
if (shouldDeleteCurrentNode) {
delete node.children[char];
return Object.keys(node.children).length === 0;
}
return false;
};
deleteHelper(this.root, word, 0);
}
Search
Searching for a string in a trie involves traversing the trie according to the characters of the string. If all characters are found and the last node is marked as the end of a word, the string exists in the trie.
search(word) {
let node = this.root;
for (const char of word) {
if (!node.children[char]) return false;
node = node.children[char];
}
return node.isEndOfWord;
}
Applications of Tries
Tries are particularly useful in various applications, including:
- Autocomplete systems: Tries can efficiently suggest completions for a given prefix.
- Spell checking: Tries can be used to quickly check if a word exists in a dictionary.
- IP routing: Tries can be used to store routing tables in networking.
Sample Interview Questions on Tries
- What are the advantages of using a trie over a hash table?
- How would you implement a trie for spell checking?
- Can you explain how tries can be used for prefix matching?
How to implement a trie?
The implementation of a trie involves creating a node structure that contains a map of children and a boolean to indicate the end of a word. The main trie class will manage the root node and provide methods for insertion, deletion, and searching.
How to use a trie for autocomplete?
To implement an autocomplete feature using a trie, follow these steps:
- Insert all possible words into the trie.
- When a user types a prefix, traverse the trie according to the characters of the prefix.
- Once the end of the prefix is reached, perform a depth-first search (DFS) from that node to collect all words that start with the prefix.
autocomplete(prefix) {
let node = this.root;
for (const char of prefix) {
if (!node.children[char]) return [];
node = node.children[char];
}
const results = [];
const findWords = (node, currentWord) => {
if (node.isEndOfWord) results.push(currentWord);
for (const char in node.children) {
findWords(node.children[char], currentWord + char);
}
};
findWords(node, prefix);
return results;
}
Practical Tips for Data Structure Interviews
How to Approach Data Structure Problems
When faced with data structure problems during an interview, it's essential to adopt a systematic approach. Here’s a step-by-step guide to help you navigate through these challenges effectively:
-
Understand the Problem:
Before diving into coding, take a moment to read the problem statement carefully. Ensure you understand what is being asked. Clarify any ambiguities with the interviewer. Ask questions like:
- What are the input and output formats?
- Are there any constraints on the input data?
- What is the expected time complexity for the solution?
-
Identify the Data Structure:
Once you understand the problem, think about which data structure would be most suitable. Consider the operations you need to perform (insertion, deletion, searching) and choose accordingly. For example:
- If you need fast lookups, a hash table might be ideal.
- If you need to maintain order, consider using a linked list or a tree.
- If you need to handle a dynamic set of elements, a dynamic array or a balanced tree could be appropriate.
-
Plan Your Solution:
Before coding, outline your approach. This could be in the form of pseudocode or a flowchart. Planning helps you visualize the solution and reduces the chances of errors. Discuss your plan with the interviewer to ensure you’re on the right track.
-
Write the Code:
Once you have a clear plan, start coding. Keep your code clean and organized. Use meaningful variable names and add comments where necessary. This not only helps you but also makes it easier for the interviewer to follow your thought process.
-
Test Your Solution:
After writing the code, test it with various cases, including edge cases. For example, if you’re working with a linked list, consider testing with:
- An empty list
- A list with one element
- A list with multiple elements
- A list with duplicate values
Explain your test cases to the interviewer and why you chose them.
-
Optimize if Necessary:
If time permits, discuss potential optimizations. Analyze the time and space complexity of your solution and suggest improvements if applicable. This shows your depth of understanding and ability to think critically.
Common Mistakes to Avoid
Interviews can be stressful, and it’s easy to make mistakes. Here are some common pitfalls to watch out for:
-
Skipping the Planning Stage:
Jumping straight into coding without a plan can lead to confusion and errors. Always take the time to outline your approach first.
-
Ignoring Edge Cases:
Failing to consider edge cases can result in incomplete solutions. Always think about how your code will handle unusual or extreme inputs.
-
Not Communicating:
Communication is key in interviews. Failing to explain your thought process can leave the interviewer in the dark. Talk through your reasoning as you work through the problem.
-
Overcomplicating Solutions:
Sometimes, the simplest solution is the best. Avoid overengineering your solution. Stick to straightforward approaches unless a more complex one is warranted.
-
Neglecting Time and Space Complexity:
Understanding the efficiency of your solution is crucial. Always analyze the time and space complexity and be prepared to discuss it with the interviewer.
Time and Space Complexity Analysis
Understanding time and space complexity is vital for evaluating the efficiency of your algorithms. Here’s a breakdown of the concepts:
Time Complexity
Time complexity measures the amount of time an algorithm takes to complete as a function of the length of the input. It is often expressed using Big O notation, which classifies algorithms according to their worst-case or upper bound performance. Here are some common time complexities:
- O(1) - Constant Time: The execution time remains constant regardless of the input size. Example: Accessing an element in an array by index.
- O(log n) - Logarithmic Time: The execution time grows logarithmically as the input size increases. Example: Binary search in a sorted array.
- O(n) - Linear Time: The execution time grows linearly with the input size. Example: Finding an element in an unsorted array.
- O(n log n) - Linearithmic Time: Common in efficient sorting algorithms like mergesort and heapsort.
- O(n2) - Quadratic Time: The execution time grows quadratically with the input size. Example: Bubble sort or selection sort.
- O(2n) - Exponential Time: The execution time doubles with each additional element in the input. Example: Solving the Fibonacci sequence using naive recursion.
Space Complexity
Space complexity measures the amount of memory an algorithm uses in relation to the input size. Like time complexity, it is also expressed in Big O notation. Here are some key points:
- O(1) - Constant Space: The algorithm uses a fixed amount of space regardless of the input size. Example: Swapping two variables.
- O(n) - Linear Space: The algorithm uses space proportional to the input size. Example: Storing elements in an array or a list.
- O(n2) - Quadratic Space: The algorithm uses space proportional to the square of the input size. Example: Creating a 2D array for dynamic programming solutions.
When discussing your solution, always mention both time and space complexity. This demonstrates your understanding of algorithm efficiency and helps the interviewer gauge the scalability of your solution.
Resources for Further Learning
To excel in data structure interviews, continuous learning is essential. Here are some valuable resources to enhance your understanding:
-
Books:
- “Introduction to Algorithms” by Thomas H. Cormen et al. - A comprehensive guide to algorithms and data structures.
- “Data Structures and Algorithms Made Easy” by Narasimha Karumanchi - A practical approach to understanding data structures and algorithms.
- “Cracking the Coding Interview” by Gayle Laakmann McDowell - A must-read for interview preparation, covering a wide range of topics.
-
Online Courses:
- Coursera - Data Structures and Algorithms Specialization - A series of courses covering fundamental concepts.
- Udacity - Data Structures and Algorithms Nanodegree - A comprehensive program with hands-on projects.
-
Practice Platforms:
- LeetCode - A popular platform for practicing coding problems, including data structures.
- HackerRank - Offers challenges and tutorials on various data structures.
- Codewars - A gamified platform for coding challenges that helps improve your skills.
By utilizing these resources and following the tips outlined above, you can significantly improve your chances of success in data structure interviews. Remember, practice is key, so dedicate time to solving problems and refining your understanding of data structures.
Key Takeaways
- Understanding Data Structures: Familiarize yourself with the fundamental concepts of data structures, including their types and importance in programming and technical interviews.
- Practice Common Operations: Master the basic operations (insertion, deletion, traversal) for arrays, linked lists, stacks, queues, trees, and graphs, as these are frequently tested in interviews.
- Focus on Sample Questions: Review and practice sample interview questions for each data structure type to build confidence and improve problem-solving skills.
- Time and Space Complexity: Develop a strong understanding of time and space complexity to analyze the efficiency of your solutions during interviews.
- Advanced Structures: Explore advanced data structures like heaps and tries, and understand their applications and operations, as they can set you apart from other candidates.
- Practical Tips: Approach problems methodically, avoid common mistakes, and utilize resources for further learning to enhance your preparation.
By mastering these key areas, you will be well-equipped to tackle data structure interview questions effectively. Remember, consistent practice and a solid understanding of concepts are crucial for success in technical interviews.
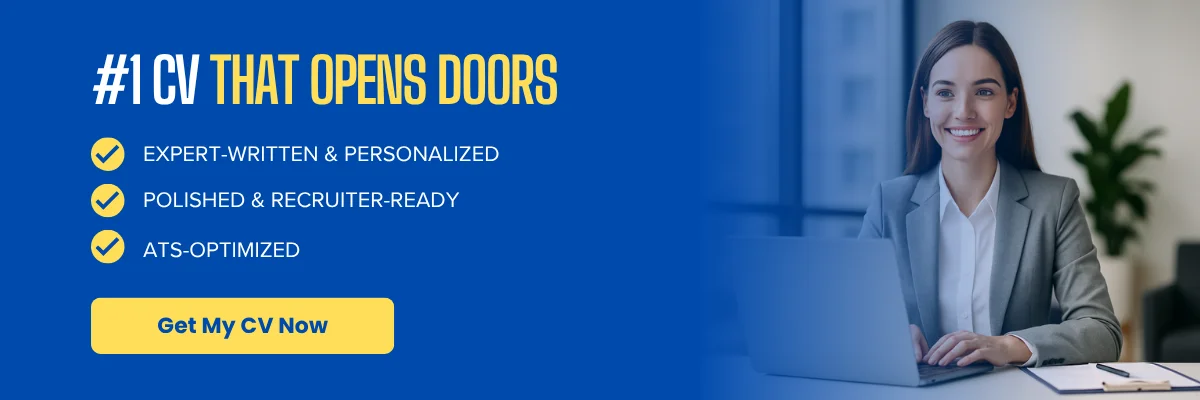
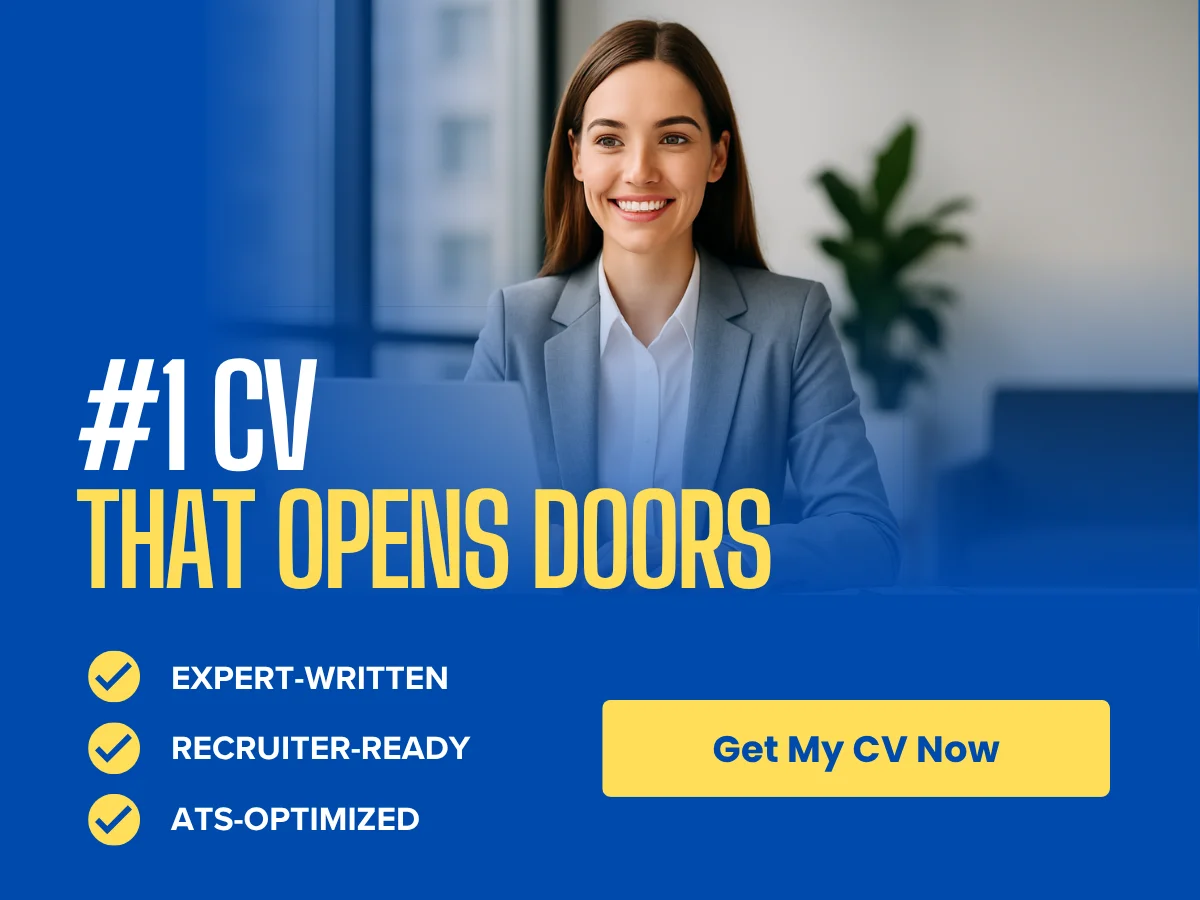